Github Actions
On this page
- What Are GitHub Actions?
- Workflow syntax for GitHub Actions
- Test GitHub Actions locally
- Debug a Github Actions' secrets
- Example
- Action composition
- Useful Github Actions
- References
What Are GitHub Actions?
GitHub Actions allow you to run arbitrary code in response to events. Events are activities that happen on GitHub such as:
- Opening a pull request
- Making an issue comment
- Labeling an issue
- Creating a new branch โฆ and many more
name: ๐ Deployon:push:branches:- masterjobs:deploy_source:name: build and deploy lambdastrategy:matrix:node-version: [18.x]runs-on: ubuntu-lateststeps:- uses: actions/checkout@v2- name: Use Node.js ${{ matrix.node-version }}uses: actions/setup-node@v1.4.2with:node-version: ${{ matrix.node-version }}- name: Install dependencies, Build application and Zip dist folder contentsrun: yarn install && zip -qq -r -j ./bundle.zip ./test/*- name: deploy zip to aws lambdauses: appleboy/lambda-action@masterwith:aws_access_key_id: ${{ secrets.AWS_ACCESS_KEY_ID }}aws_secret_access_key: ${{ secrets.AWS_SECRET_ACCESS_KEY }}aws_region: ${{ secrets.AWS_REGION }}function_name: kan_testzip_file: bundle.zip
Workflow syntax for GitHub Actions
Workflow files use YAML syntax, and must have either a .yml or .yaml file extension. If you're new to YAML and want to learn more, see "Learn YAML in five minutes."
name: The name of your workflow.
on(required): The name of the GitHub event that triggers the workflow. You can provide a single event string
, array
of events, array
of event types
Example using a single event
# Trigger on pushon: push
Example using a list of events
# Trigger the workflow on push or pull requeston: [push, pull_request]
Example using multiple events with activity types or configuration
on:# Trigger the workflow on push or pull request,# but only for the master branchpush:branches:- master- "releases/**"paths:- "**.js"pull_request:branches:- master# Also trigger on page_build, as well as release created eventspage_build:release:types: # This configuration does not affect the page_build event above- createdschedule:# * is a special character in YAML so you have to quote this string- cron: "*/15 * * * *"
crontab guru: The quick and simple editor for cron schedule expressions
Some helpful patterns
// Every Monday at 1PM UTC (9AM EST)0 13 * * 1// At the end of every day0 0 * * *// Every 10 minutes*/10 * * * *
jobs: A workflow run is made up of one or more jobs. Jobs run in parallel by default. You can run an unlimited number of jobs as long as you are within the workflow usage limits. For more information, see "Usage limits."
jobs:my_first_job:name: My first jobmy_second_job:name: My second job
Identifies any jobs that must complete successfully before this job will run.
jobs:job1:job2:needs: job1job3:needs: [job1, job2]
runs-on: The type of machine to run the job on. The machine can be either a GitHub-hosted runner, or a self-hosted runner.
runs-on: ubuntu-latest
steps: A job contains a sequence of tasks called steps.
- Steps can run commands, run setup tasks, or run an action in your repository, a public repository, or an action published in a Docker registry.
- Not all steps run actions, but all actions run as a step.
- Run unlimited number of steps as long as you are within the workflow usage limits
steps:- name: My first stepif: ${{ github.event_name == 'pull_request' && github.event.action == 'unassigned' }}run: echo This event is a pull request that had an assignee removed.
uses: Selects an action to run as part of a step in your job.
- An action is a reusable unit of code.
- You can use an action defined in the same repository as the workflow, a public repository, or in a published Docker container image.
steps:- name: My first step# Uses the master branch of a public repositoryuses: actions/heroku@master- name: My second step# Uses a specific version tag of a public repositoryuses: actions/aws@v2.0.1
with: A map of the input parameters defined by the action.
- Each input parameter is a key/value pair.
- Input parameters are set as environment variables.
- The variable is prefixed with INPUT and converted to upper case.
jobs:my_first_job:steps:- name: My first stepuses: actions/hello_world@masterwith:first_name: Monamiddle_name: Thelast_name: Octocat
Test GitHub Actions locally
When you run act it reads in your GitHub Actions from .github/workflows/ and determines the set of actions that need to be run. It uses the Docker API to either pull or build the necessary images, as defined in your workflow files and finally determines the execution path based on the dependencies that were defined. Once it has the execution path, it then uses the Docker API to run containers for each action based on the images prepared earlier. The environment variables and filesystem are all configured to match what GitHub provides.
brew install act# list all workflowsact -l# dry run the specific workflow, check syntax errorsact -j build -nact --secret-file act.secretsact -r -j pr_log -P ubuntu-latest=catthehacker/ubuntu:act-latest
Debug a Github Actions' secrets
echo ${{secrets.YOUR_SECRET }} | sed 's/./& /g'
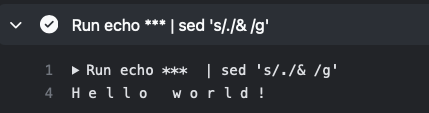
This would output the secret with spaces between each character, Github will not recognize the secret and will not hide it.
! Beware that the secret will then be in the actions logs in clear form, and everybody having access to the repo will be able to see it.
sed
is a stream editor,s
: substitution,.
: match anything which is greedy,/g
: global replacement
Example
Deploy your projects to Github Pages
name: ๐ Build and Deployon:push:branches:- masterbuild-and-deploy:runs-on: ubuntu-lateststeps:- name: Checkout ๐๏ธuses: actions/checkout@v2 # If you're using actions/checkout@v2 you must set persist-credentials to false in most cases for the deployment to work correctly.with:persist-credentials: false# fetch-depth: '0' # fetch the entire git history:- name: Install and Build ๐ง # This example project is built using npm and outputs the result to the 'build' folder. Replace with the commands required to build your project, or remove this step entirely if your site is pre-built.run: |yarn installyarn run build- name: Deploy ๐uses: JamesIves/github-pages-deploy-action@releases/v3with:GITHUB_TOKEN: ${{ secrets.GITHUB_TOKEN }}BRANCH: gh-pages # The branch the action should deploy to.FOLDER: build # The folder the action should deploy.
outputs
GitHub Actions now allows you to take step outputs and output them into other jobs. Now if you have a job that is dependent on some data from another job, instead of needing to save it in a file and upload an Artifact, you can just use Job Outputs and save just the data itself with minimal encoding:
Step outputs
on:push:branch:- '*'jobs:test_strings:name: test stringsruns-on: ubuntu-lateststeps:- name: create stringrun: |MY_STRING="hello world"echo "::set-output name=content::$MY_STRING"id: my_string- name: display stringrun: |echo "The string is: ${{ steps.my_string.outputs.content }}"
Job Outputs
name: Do thingson: pushjobs:job1:runs-on: ubuntu-latestoutputs:url: ${{ steps.step1.outputs.url }} # map step output to job outputsteps:- id: step1name: send url to other jobrun: echo "::set-output name=url::https://google.com"job2:runs-on: ubuntu-latestneeds: job1steps:- run: user/some-action@v1with:url: ${{ needs.job1.outputs.url }} # grab job output here
Action composition
Reference other actions to reduce duplication in your workflows.
Using Composite GitHub Actions to make your Workflows smaller and more reusable
Useful Github Actions
- Keepalive Workflow:GitHub action to prevent GitHub from suspending your cronjob based triggers due to repository inactivity
- GitHub Pages Deploy Action:This GitHub Action will automatically deploy your project to GitHub Pages. It can be configured to push your production-ready code into any branch you'd like, including gh-pages and docs.
- AWS cli install action:Action to install the most recent version of the AWS-CLI
- HashiCorp - Setup Terraform:The hashicorp/setup-terraform action is a JavaScript action that sets up Terraform CLI in your GitHub Actions workflow.
- fastpages:An easy to use blogging platform, with support for Jupyter notebooks, Word docs, and Markdown.
- Release Drafter:Drafts your next release notes as pull requests are merged into master.
- cache:Cache dependencies and build outputs in GitHub Actions
- Auto Assign Reviewer By Issuer:A GitHub Action to automatically assigns reviewer by issuer
- Condition based Pull Request Labeler:Implements a GitHub Action that labels Pull Requests based on configurable conditions.It is inspired by the example Pull Request Labeller, but intends to provide a richer set of options.